지난 포스팅에 회원가입까지 구현을 했고 이번엔 회원목록조회를 구현해보겠습니다.
제시된 이미지를 보며 만들어봅시당
일단 DB에 있는 정보를 가져오는 메서드를 만들어주고,
[MemberListViewCommend]
뚝딱뚝딱 커맨드를 만들어줍니다.
JSP에서 lists 값을 가져와서 출력해야 하기 때문에 request.setAttribute("lists", lists) 를 해줍시다.
컨트롤러에 memberListView.do에 대한 요청을 추가해주고, JSP가 잘 연결되어 출력되는지 확인해줍니다.
[MemberListView.jsp]
<%@page import="org.project.MemberDto"%>
<%@page import="java.util.ArrayList"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
ArrayList<MemberDto> lists=(ArrayList<MemberDto>)request.getAttribute("lists");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MemberList</title>
<link rel="stylesheet" href="css/memberListView.css" />
</head>
<body>
<%@include file="header.jsp" %>
<div class="container">
<div class="inner-con">
<table>
<thead>
<tr>
<th colspan="7" class="t1">회원목록조회/수정</th>
</tr>
<tr>
<th>회원번호</th>
<th>회원성명</th>
<th>전화번호</th>
<th>주소</th>
<th>가입일자</th>
<th>고객등급</th>
<th>거주지역</th>
</tr>
</thead>
<tbody>
<%
for (MemberDto list:lists) {
%>
<tr>
<td><a href="memberView.do?custNo=<%=list.getCustNo() %>"><%=list.getCustNo() %></a></td>
<td><%=list.getCustName() %></td>
<td><%=list.getPhone() %></td>
<td><%=list.getAddress() %></td>
<td><%=list.getJoinDate() %></td>
<td><%=list.getGrade() %></td>
<td><%=list.getCity() %></td>
<%
}
%>
</tr>
</tbody>
<tfoot></tfoot>
</table>
</div>
</div>
<%@include file="footer.jsp" %>
</body>
</html>
/*memberListView.css*/
@import url("reset.css");
@import url("header.css");
@import url("footer.css");
.container {
width: 100%;
height: calc(100vh - 130px - 50px);
background-color: #e9e9e9;
}
.inner-con {
display: flex;
justify-content: center;
}
.t1 {
line-height: 80px;
font-size: 1.5em;
border: none;
}
table {
text-align: center;
}
table thead tr th {
line-height: 50px;
border: 3px double #c0c0c0;
padding: 0 20px;
}
table tbody tr td {
border: 3px double #c0c0c0;
padding: 0 20px;
}
table tbody tr td a {
color: deepskyblue;
}
<%
ArrayList<MemberDto> lists=(ArrayList<MemberDto>)request.getAttribute("lists");
%>
lists를 받아와서 데이터를 출력합니당
그리고 이어지는 회원정보수정에 나오겠지만 회원번호를 클릭하면 회원정보수정하는 페이지로 연결되게 해야해서 회원번호에는 a태그를 추가했습니다.
회원정보수정은 다음 포스팅에서 이어집니다!
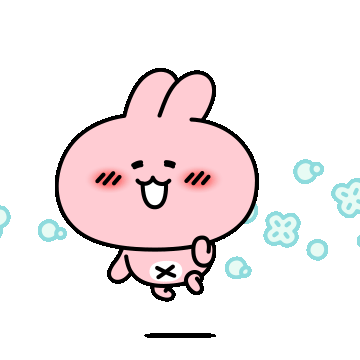
다음으론 회원매출조회 페이지를 만들어보겠습니다.
참고하여 DTO를 하나 더 만들어줍시다
[MoneyDto]
Dao에 매출을 조회하는 메서드를 추가해줍니다.
join을 이용해서 쿼리문을 작성해줍니다.
이렇게 긴 쿼리문일수록 cmd에서 정상적으로 출력되는지 확인한 후에 작성해야 합니다.
쿼리문 제외하고는 회원목록조회와 비슷하기 때문에 이후엔 어렵지않게 작성할 수 있을겁니다.
[MemberMoneyViewCommend]
[memberMoneyView.jsp]
<%@page import="java.util.ArrayList"%>
<%@page import="org.project.MoneyDto"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
ArrayList<MoneyDto> moneys =
(ArrayList<MoneyDto>)request.getAttribute("moneys");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MoneyView</title>
<link rel="stylesheet" href="css/memberMoneyView.css" />
</head>
<body>
<%@include file="header.jsp" %>
<div class="container">
<div class="inner-con">
<table>
<thead>
<tr>
<th colspan="4" class="t1">회원매출조회</th>
</tr>
<tr>
<th>회원번호</th>
<th>회원성명</th>
<th>고객등급</th>
<th>매출</th>
</tr>
</thead>
<tbody>
<%
for (MoneyDto list:moneys) {
%>
<tr>
<td><%=list.getCustNo() %></td>
<td><%=list.getCustName() %></td>
<td><%=list.getGrade() %></td>
<td><%=list.getPrice() %></td>
<%
}
%>
</tr>
</tbody>
<tfoot></tfoot>
</table>
</div>
</div>
<%@include file="footer.jsp" %>
</body>
</html>
/*memberMoneyView.css*/
@import url("reset.css");
@import url("header.css");
@import url("footer.css");
.container {
width: 100%;
height: calc(100vh - 130px - 50px);
background-color: #e9e9e9;
}
.inner-con {
display: flex;
justify-content: center;
}
.t1 {
line-height: 80px;
font-size: 1.5em;
border: none;
}
table {
text-align: center;
}
table thead tr th {
line-height: 50px;
border: 3px double #c0c0c0;
padding: 0 20px;
}
table tbody tr td {
border: 3px double #c0c0c0;
padding: 0 20px;
}
회원목록조회와 같은 방법으로 출력해주시면 됩니다.
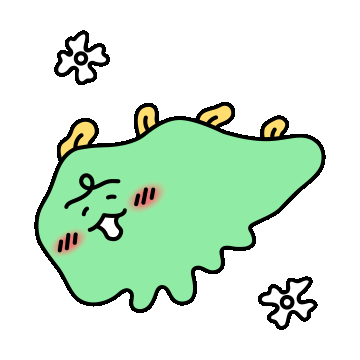
이렇게 회원목록과 매출조회하는 리스트 두개를 출력해 보았습니다.
다음번 포스팅에선 마지막으로 회원정보 수정하기를 구현해 보겠습니다!
'[자기계발] > 자격증' 카테고리의 다른 글
[과정평가형 정보처리기능사] 22년도 4회차 실기 합격! 후기😒 (0) | 2022.10.12 |
---|---|
[과정평가형 정보처리산업기사] 실기 예제 - 쇼핑몰 회원관리 프로그램(3) 회원정보수정 구현하기 (0) | 2022.08.19 |
[과정평가형 정보처리산업기사] 실기 예제 - 쇼핑몰 회원관리 프로그램(1) 회원가입 구현하기(유효성 검사, 회원번호 자동생성) (0) | 2022.08.19 |